Parameterize: CHAIR
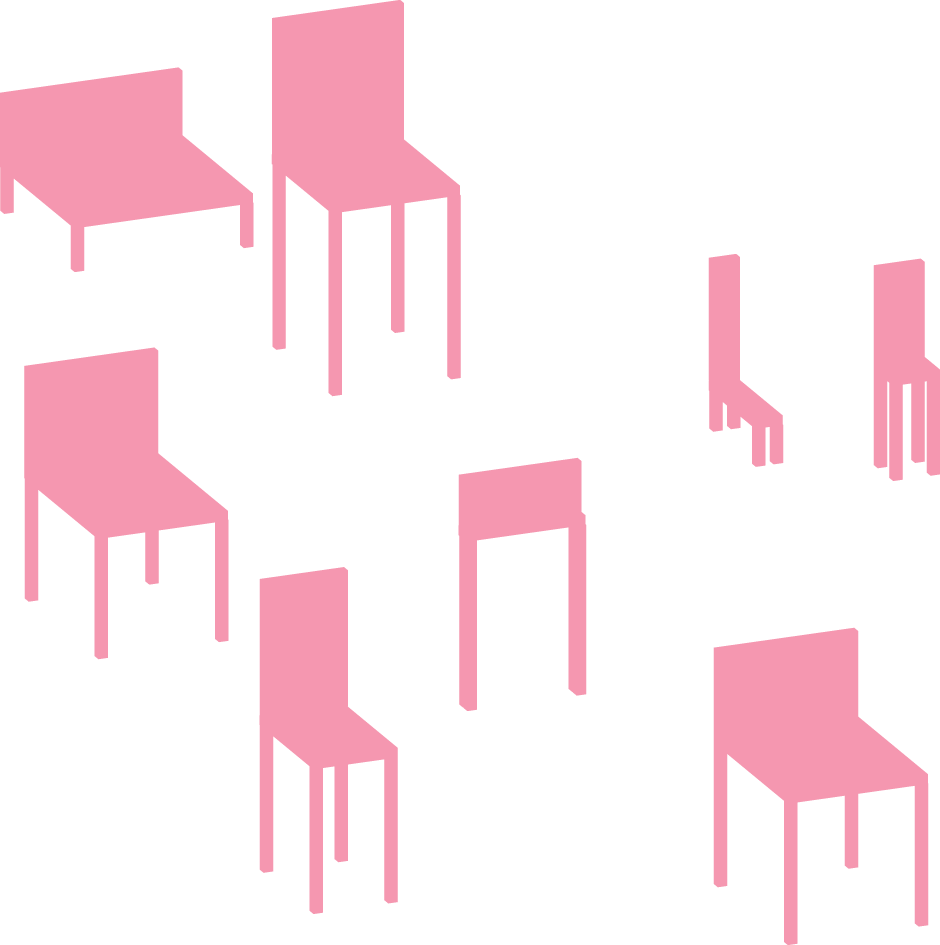
The first step in creating a parameterized design is to decompose the form into variables. A simple, parameterized chair has variables for the dimensions of the seat, the height of the back, and the thickness of the frame. One unique chair exists for each set of variable values. The design space is the range of all possible chairs that can be generated within the system.
Once the parameters have been identified, they can be used to draw the chair, and after a value has been set for each variable, the code responsible for translating those values into lines and shapes will display the chair on-screen. Choosing values for each parameter at random is one way to explore unexpected chairs within the design space.
/**
* Parameterize: Chair
* from Form+Code in Design, Art, and Architecture
* by Casey Reas, Chandler McWilliams, and LUST
* Princeton Architectural Press, 2010
* ISBN 9781568989372
*
* This code was written for Processing 1.2+
* Get Processing at http://www.processing.org/download
*/
int chairSeatHeight = 100;
int chairWidth = 50;
int chairDepth = 50;
int chairBackHeight = 100;
int chairFrameThickness = 10;
void setup() {
size(1024, 1024, P3D);
smooth();
fill(0);
stroke(0);
noLoop();
}
void draw() {
background(255);
ortho(0, width, 0, height, 0, 300);
pushMatrix();
translate(width, height);
rotateX(-PI / 9);
rotateY(PI / 8);
scrambleChair();
drawChair();
popMatrix();
}
void drawChair() {
// back
pushMatrix();
translate(chairWidth/2, chairBackHeight/2);
box(chairWidth, chairBackHeight, chairFrameThickness);
popMatrix();
// seat
pushMatrix();
translate(chairWidth/2, chairBackHeight + chairFrameThickness/2, chairDepth/2 - chairFrameThickness/2);
box(chairWidth, chairFrameThickness, chairDepth);
popMatrix();
// legs
pushMatrix();
translate(chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, 0);
box(chairFrameThickness, chairSeatHeight, chairFrameThickness);
popMatrix();
pushMatrix();
translate(chairWidth - chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, 0);
box(chairFrameThickness, chairSeatHeight, chairFrameThickness);
popMatrix();
pushMatrix();
translate(chairWidth - chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, chairDepth - chairFrameThickness);
box(chairFrameThickness, chairSeatHeight, chairFrameThickness);
popMatrix();
pushMatrix();
translate(chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, chairDepth - chairFrameThickness);
box(chairFrameThickness, chairSeatHeight, chairFrameThickness);
popMatrix();
}
void scrambleChair() {
chairSeatHeight = floor(random(10, 200));
chairWidth = floor(random(10, 200));
chairDepth = floor(random(10, 200));
chairBackHeight = floor(random(10, 200));
}
void mousePressed() {
redraw();
}
Contributed Examples
-
OpenFrameworks
/** * Parameterize: Chair from Form+Code in Design, Art, and Architecture * implemented in OpenFrameworks by Anthony Stellato <http://rabbitattack.com/> * * Requires OpenFrameworks available at http://openframeworks.cc/ * * For more information about Form+Code visit http://formandcode.com */ // =========================================================== // - Parameterize_Chair.h // =========================================================== #include "ofMain.h" class testApp : public ofBaseApp{ public: void setup(); void draw(); void drawBox(int size); void drawBox(int w, int h, int d); void scrambleChair(); void drawChair(); void mousePressed(int x, int y, int button); }; // =========================================================== // - Parameterize_Chair.cpp // =========================================================== #include "Parameterize_Chair.h" int chairSeatHeight = 100; int chairWidth = 50; int chairDepth = 50; int chairBackHeight = 100; int chairFrameThickness = 10; //-------------------------------------------------------------- void testApp::setup(){ ofSetFrameRate(60); ofEnableSmoothing(); } //-------------------------------------------------------------- void testApp::draw(){ ofBackground(255, 255, 255); ofSetColor(0, 0, 0); ofPushMatrix(); ofTranslate(ofGetWidth()/2, ofGetHeight()/2, 0); ofRotate(ofRadToDeg(-PI/9), 1, 0, 0); ofRotate(ofRadToDeg(PI/8), 0, 1, 0); drawChair(); ofPopMatrix(); } void testApp::drawChair(){ //back ofPushMatrix(); ofTranslate(chairWidth/2, chairBackHeight/2, 0); drawBox(chairWidth, chairBackHeight, chairFrameThickness); ofPopMatrix(); //seat ofPushMatrix(); ofTranslate(chairWidth/2, chairBackHeight + chairFrameThickness/2, chairDepth/2 - chairFrameThickness/2); drawBox(chairWidth, chairFrameThickness, chairDepth); ofPopMatrix(); //legs ofPushMatrix(); ofTranslate(chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, 0); drawBox(chairFrameThickness, chairSeatHeight, chairFrameThickness); ofPopMatrix(); ofPushMatrix(); ofTranslate(chairWidth - chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, 0); drawBox(chairFrameThickness, chairSeatHeight, chairFrameThickness); ofPopMatrix(); ofPushMatrix(); ofTranslate(chairWidth - chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, chairDepth - chairFrameThickness); drawBox(chairFrameThickness, chairSeatHeight, chairFrameThickness); ofPopMatrix(); ofPushMatrix(); ofTranslate(chairFrameThickness/2, chairBackHeight + chairSeatHeight/2 + chairFrameThickness, chairDepth - chairFrameThickness); drawBox(chairFrameThickness, chairSeatHeight, chairFrameThickness); ofPopMatrix(); } void testApp::scrambleChair(){ chairSeatHeight = floor(ofRandom(10, 200)); chairWidth = floor(ofRandom(10, 200)); chairDepth = floor(ofRandom(10, 200)); chairBackHeight = floor(ofRandom(10, 200)); } void testApp::drawBox(int size){ drawBox(size, size, size); } void testApp::drawBox(int w, int h, int d){ float x1 = -w/2.f; float x2 = w/2.f; float y1 = -h/2.f; float y2 = h/2.f; float z1 = -d/2.f; float z2 = d/2.f; glBegin(GL_QUADS); // front glNormal3d(0, 0, 1); glVertex3f(x1, y1, z1); glVertex3f(x2, y1, z1); glVertex3f(x2, y2, z1); glVertex3f(x1, y2, z1); // right glNormal3d(1, 0, 0); glVertex3f(x2, y1, z1); glVertex3f(x2, y1, z2); glVertex3f(x2, y2, z2); glVertex3f(x2, y2, z1); // back glNormal3d(0, 0, -1); glVertex3f(x2, y1, z2); glVertex3f(x1, y1, z2); glVertex3f(x1, y2, z2); glVertex3f(x2, y2, z2); // left glNormal3d(-1, 0, 0); glVertex3f(x1, y1, z2); glVertex3f(x1, y1, z1); glVertex3f(x1, y2, z1); glVertex3f(x1, y2, z2); // top glNormal3d(0, 1, 0); glVertex3f(x1, y1, z2); glVertex3f(x2, y1, z2); glVertex3f(x2, y1, z1); glVertex3f(x1, y1, z1); // bottom glNormal3d(0, -1, 0); glVertex3f(x1, y2, z1); glVertex3f(x2, y2, z1); glVertex3f(x2, y2, z2); glVertex3f(x1, y2, z2); glEnd(); } //-------------------------------------------------------------- void testApp::mousePressed(int x, int y, int button){ scrambleChair(); }
We are looking for implementations of the code examples in other programming languages to post on the site. If you would like to submit a sample, or if you find a bug, please write to